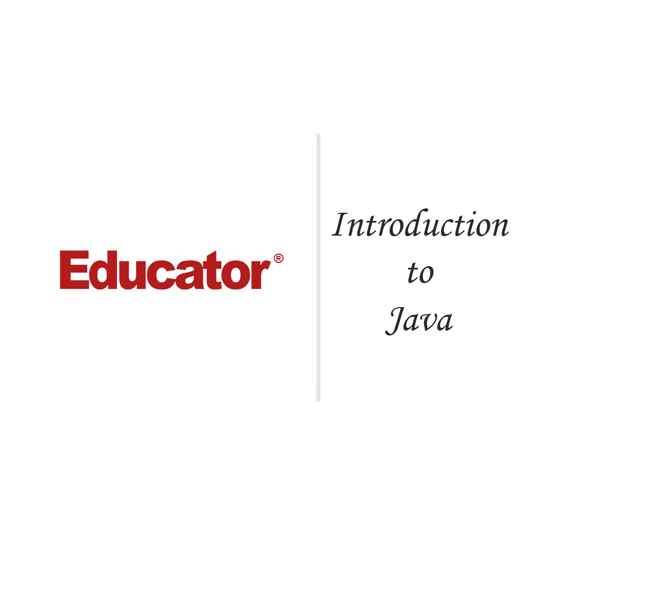
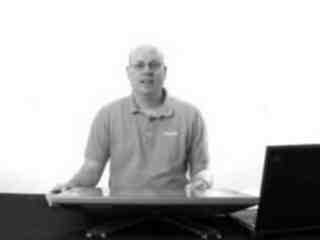
Maury Hillstrom
Introduction to Java
Slide Duration:Table of Contents
Section 1: Java
Introduction to Java
31m 13s
- Intro0:00
- What is Java?0:12
- Definition of Java0:13
- Writing a Java Program3:48
- Example: Converter IO Program3:49
- Example: Grade Program8:00
- Example: Writing a Java Program12:13
- Starting Point of All Java Code14:00
- 'Public Static Void Main'14:01
- 'Public Static Void Main' Syntax16:07
- Syntax16:08
- String16:27
- args16:41
- How to Add Comments17:13
- How to Add and Use Comments17:14
- Java Statements17:59
- Java Statements and Example18:00
- Example1: Text-printing Program18:44
- Example 2: Text-printing on Multiple Lines20:12
- Example 3: Addition Program that Displays the Sum of Two Numbers21:11
- Example 4: Program that Determines Calculations of Two Numbers24:43
- Example 5: Running Programs Through NetBeans IDE25:50
Working with Java
31m 57s
- Intro0:00
- History of Java0:09
- History of Java0:10
- Requirements of Java2:35
- Free to Download & Install2:36
- System's Path Environment Variable4:40
- Java's Two-Step Process5:48
- Create Source File5:49
- Compile Source File Into Bytecode6:49
- Java Application Creation7:35
- Two Main Ways to Create Java Application7:36
- Other Popular Java-compatible IDE8:08
- Importing Classes8:45
- Importing Classes, Example, and Syntax8:46
- Arithmetic Rules of Precedence10:02
- PEMDAS vs. P(MDR)(AD)(Java)10:03
- Four More Program Examples13:22
- Example: Circle Geometry14:06
- Example: Searching Through a Text String19:50
- Example: Doing a Find & Replace23:35
- Example: Calendar Functions25:45
Classes, Objects, and Methods
29m 34s
- Intro0:00
- Classes, Objects, and Methods0:09
- Class0:10
- Object0:57
- Attributes1:14
- Method1:33
- Arguments1:48
- Instance Variables2:17
- Instance Variables2:18
- Set and Get Methods3:15
- Set and Get Methods3:16
- Primitive Types vs. Reference Types4:11
- Primitive Types4:12
- Reference Type6:56
- Constructors7:22
- Attributes of Constructors7:23
- Example 1: Prints a Table of Squares and Cubes From 0 to 108:26
- Example 2: Bank Account Class with Constructor11:54
- Example 3: Create and Change Account Object14:14
- Example 4: Bank Account Debit Function18:44
- Example 5: Debit Account Balance Program20:08
- Example 6: Hardware Store Inventory & Invoice22:50
- Example 7: Hardware Store Inventory & Invoice Main Class26:05
If Logic
34m 20s
- Intro0:00
- The Mighty 'If'0:08
- If Logic and If Statements0:09
- Conditional Statements in Java1:44
- If1:45
- If…Else2:07
- If…Else If….Else2:33
- Switch3:15
- Pseudocode3:41
- Pseudocode3:42
- Examples4:05
- Compound Assignment Operators6:17
- Compound Assignment Operators and Examples6:18
- Boolean Logical Operators9:02
- && Operator9:03
- | | Operator9:58
- ! Operator11:38
- Example 1: Program that Determines the Larger of Two Numbers13:00
- Example 2: Determine the Largest and Smallest Entry15:55
- Example 3: Determine if a Number is Odd or Even19:24
- Example 4: Determine if Three Values Could Form a Triangle21:44
- Example 5: Run Application for Triangle24:23
- Example 6: Basic If…Else Statement25:11
- Example 7: Basic If…Else If…Else Statement26:52
- Example 8: Catch Possibilities if User Enters Invalid Numbers28:38
- Example 9: Basic Switch Statement31:10
Loops
37m
- Intro0:00
- Types of Loops0:07
- For Loop0:54
- While Loop1:15
- Do…While Loop1:39
- Increment and Decrement Operators2:26
- Operators Overview3:11
- Pre-Increment4:34
- Pre-Decrement5:35
- Post-Increment6:01
- Post-Decrement6:25
- Counters vs. Sentinels7:06
- Counters7:07
- Sentinels7:40
- Break & Continue Statements8:46
- Break8:47
- Continue9:29
- While Loop Syntax10:03
- While Loop Syntax and Example10:04
- For Loop Syntax11:54
- For Loop Syntax and Example11:55
- Example 1: Calculate Commissions for Sales Staff13:55
- Example 2: Program Calculates Average Miles Per Gallon18:56
- Example 3: Find the Smallest of Entered Numbers23:30
- Example 4: Return the Lyrics to 'The 12 Days of Christmas'27:37
- Example 5: Do While Setup31:50
- Example 6: Break Loop Statement Example34:21
- Example 7: Continue Loop Statement Example35:05
Modules
33m 56s
- Intro0:00
- Types of Modules0:09
- Methods & Classes0:10
- Packages0:28
- Static Class Members1:20
- Static Class Members1:21
- Example of Static Class Members1:51
- Queues vs. Stacks3:21
- Queues3:51
- Stacks5:06
- Push and Pop5:42
- Method Overloading6:05
- Method Overloading6:06
- The 'this' Keyword7:13
- 'this' Keyword7:14
- Example7:28
- Composition vs. Inheritance8:05
- Composition and Example8:23
- Inheritance and Example9:33
- Example 1: Roll a Six-diced Die 10,000 Times10:53
- Example 2: Program Calculates Charges for Parking Lot14:47
- Example 3: Test Application for Class Parking20:30
- Example 4: Program that Converts Fahrenheit to Celsius and Vice Versa23:43
- Example 5: Program Simulates Tossing a Coin29:25
Arrays
34m 3s
- Intro0:00
- What is an Array?0:08
- Attributes of Array0:09
- Types of Arrays1:10
- Single-Dimensional1:18
- Two-Dimensional2:01
- How to Declare an Array3:15
- Declaring an Array: Single Dimension3:16
- Declaring an Array: Two Dimension4:12
- The Enhanced 'for' Statement5:02
- 'for' Statement5:03
- Passing Arrays to Methods7:14
- Pass by Value7:34
- Pass by Reference7:54
- Example 1: Simple Array8:24
- Example 2: Program Simulates Rolling Two Six-sided Dice 36,000 Times9:54
- Example 3: Application Roll Two Dice14:49
- Example 4: Program Totals Sales for Sales Staff and Products17:24
- Example 5: Application for Class Sales21:08
- Example 6: Card Class Represents a Playing Card24:43
- Example 7: Deck Class Represents a Deck of Playing Cards26:36
- Example 8: Card Shuffling and Dealing Application30:35
Inheritance
28m 15s
- Intro0:00
- Composition vs. Inheritance0:07
- Composition & Example0:17
- Inheritance & Example1:16
- Composition vs. Inheritance2:04
- Visual Diagram2:05
- Inheritance Class Structure4:36
- Parent Class4:37
- Child Class4:51
- Purpose of Inheritance5:01
- Method Overriding vs. Overloading6:32
- Method Overriding6:39
- Method Overloading7:28
- Inheritance Class Hierarchy8:01
- Inheritance Class Hierarchy8:02
- Protected Members10:27
- Public, Protected, and Private10:28
- Example 1: Basic Inheritance with Constructors12:11
- Example 2: Program Exhibits Both Composition and Inheritance16:03
- Example 3: Class Point Definition21:07
- Example 4: Class Quadrilateral Definition22:15
- Example 5: Class Trapezoid Definition23:09
- Example 6: Class Parallelogram Definition24:05
- Example 7: Class Rectangle Definition24:37
- Example 8: Class Square Definition24:50
- Example 9: Main Application for Shapes Exercise25:42
Polymorphism
36m 53s
- Intro0:00
- What is Polymorphism?0:08
- Definition of Polymorphism0:09
- Polymorphism in Programming1:10
- Superclass1:11
- Subclass2:11
- Variable2:21
- Method2:31
- Polymorphism in Programming3:46
- Superclass3:47
- Subclass4:16
- Variable4:22
- Method4:31
- Abstract Classes vs. Concrete Classes6:31
- Abstract Classes6:32
- Abstract Class Polymorphism Example9:19
- Abstract Superclass9:20
- Concrete Subclasses9:58
- 'Final' Keyword for Superclass Methods13:49
- 'Final' Keyword13:50
- Example14:21
- Example 1: Program to Demonstrate Basic Polymorphism16:25
- Example 2: Program to Demonstrate Polymorphism with Multiple Subclasses18:35
- Example 3: Program Tests Shape Hierarchy25:12
- Example 4: Definition of Class Shape27:17
- Example 5: Definition of Class Two Dimensional Shape28:31
- Example 6: Definition of Class Three Dimensional Shape29:29
- Example 7: Definition of Class Sphere33:45
Exception Handling
22m 45s
- Intro0:00
- What is Exception Handling?0:09
- Definition of Exception Handling0:10
- Why Account for Errors?1:31
- Why Account for Errors?1:32
- Common Types of Errors3:51
- User Caused3:52
- Programmer or System Caused5:31
- Using the 'Try… Catch' Process7:30
- Try7:35
- Catch8:27
- Try… Catch Syntax8:51
- Try… Catch Syntax8:52
- Uncaught Exceptions9:44
- Handling Uncaught Exceptions9:45
- Throw' Statement11:03
- Throw Statement11:04
- Throw Syntax11:22
- Example 1: Program to Catch Divide by Zero Error11:41
- Example 2: Program to Demonstrate Finally Keyword16:18
- Example 3: Superclass and Subclass Exceptions19:20
Loading...
This is a quick preview of the lesson. For full access, please Log In or Sign up.
For more information, please see full course syllabus of Java
For more information, please see full course syllabus of Java
Java Introduction to Java
Lecture Description
In this lesson our instructor gives an introduction to Java. First he defines Java. Then, he talks about writing a java program, starting point of all java code, and the 'Public Static Void Main' syntax. After that, he discusses how to add comments and java statements. Five example videos round up this lesson.
Bookmark & Share
Embed
Share this knowledge with your friends!
Copy & Paste this embed code into your website’s HTML
Please ensure that your website editor is in text mode when you paste the code.(In Wordpress, the mode button is on the top right corner.)
×
- - Allow users to view the embedded video in full-size.
Next Lecture
Previous Lecture
0 answers
Post by Victoria Su on June 25, 2021
Hello,
After typing in the program shown on the slide, the code does not run. The program says that there is an error and that it cannot find the symbol in the line public static void main( Strings args[]) and more specifically the symbol for class Strings. I'm not sure why this is the case.
2 answers
Last reply by: Jianyingcheng
Sun Mar 15, 2020 11:35 AM
Post by ??&linda on December 14, 2019
How can I code Java on Windows 10?
1 answer
Last reply by: Qin Qiu
Sun Sep 25, 2022 12:24 PM
Post by Stella Sun on June 24, 2019
12:23 69 lol
0 answers
Post by S Fonseca on December 28, 2018
Hello Prof Hillstrom:
I recently viewed a set of 5 - 6 lessons from this Java course and wanted to give you (and educator.com) some feedback. First of all, you did a very nice job explaining the beginner level Java material in these lessons. Congrats!
Your presentation style is very nice and just at the right pace. You also explain the Java concepts very well.
Just a few positive recommendations are in order:
(1) It would be helpful for student learning to have the sample code from these lessons. While a student can stop the lesson and type in these programs, additional course materials providing the source code would improve student understanding. In addition, providing source code would increase interest in your course! (And instead of file-sharing, simple zipped sets of program text files would not be very large.)
(2) This course explains the Beginner and some Intermediate concepts very well. And it would be great if you also created Java II and III courses -- moving to more advanced concepts of Java (such as JavaBeans, multithreading, running C or C++ programs from within Java, web programming in Java, networking programs, Enterprise topics, etc.). This is not a recommendation only for you (but, also to educator.com) -- there are many other educator.com courses which do a great job at the beginner level, BUT do not continue into more advanced areas. Much greater interest would be created if different levels of Java were covered.
Overall, however, your Java course is very good and helpful in providing a way for students to learn Java!
Regards.
1 answer
Last reply by: Hui Lu
Thu Jun 29, 2017 11:07 AM
Post by Hui Lu on June 29, 2017
Video doesn't work?
1 answer
Sun Nov 6, 2016 11:03 PM
Post by Firebird wang on November 2, 2016
Sir, I know that AP Statistics is not your subject, but I just wonder if you are able to watch the two videos which called Practice Test 2013 AP Statistics an Practice Test 2014 AP Statistics in the AP Statistics content? Both videos showing network error, I dont know why. I already tried in different computers already.
0 answers
Post by jitendra singh on January 25, 2016
I like your digital writing can you please give the name of the computer. I am interested in buying it
thanks
1 answer
Fri May 29, 2015 7:08 PM
Post by alina trandafir on May 23, 2015
Hi professor,
Quick question can you explain a little more on what it means to return data.
I noticed wether we have data that is being input or output the method is void.
So when we put public static void main(String args [], when would it not be void?
4 answers
Last reply by: Mike Six
Wed Sep 21, 2016 11:09 PM
Post by Ihechukwudere Okoroego on April 24, 2014
Hello Professor, Those are helpful lectures. Please can you send me exercises files for all the lectures to give me more practice.
Thanks
0 answers
Post by Martha Villanueva on January 16, 2014
Maury, thanks for the lesson.
When working in NetBeans, what are the differences between "Default Package" and "Source Package"? Why the warning in NB saying is was not recommended to place java classes in the default package? Is there any best practice there that will be advisable to follow?
Thanks and regards
0 answers
Post by hawa guled on October 2, 2013
do u think you may starting basic computer training like software and hard
ware
0 answers
Post by Natalia Frenkel on April 29, 2013
When I tried to test my first java code, I got thei mwssage:
Caused by: java.lang.ClassNotFoundException: chargebac
k.MyFirstApp.java
at java.net.URLClassLoader.findClass(URLClassLoader.java:434)
at java.lang.ClassLoader.loadClass(ClassLoader.java:660)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:358)
at java.lang.ClassLoader.loadClass(ClassLoader.java:626)
Please, help me to undestand why is that. What should I fix ?
0 answers
Post by R Grose on February 11, 2013
Is there a reference or textbook you recommend to go along with the lecture
0 answers
Post by Dana Amin on December 4, 2012
I would like to know if Java is used to creat systems out there please? such as bank system and companies system ? if not what is that language which is used ? thanks
0 answers
Post by Patti Henderson on November 7, 2012
Syntax Errors - } ; ) missing
1 answer
Thu Nov 1, 2012 2:38 PM
Post by george masih on June 12, 2012
import java.io.*;
import java.util.*;
class string {
public static void main(String[] args){
Scanner sc = new Scanner(system.in);
String i = sc.next();
System.out.println(i);
System.out.print("tesing input ");
----------------
i got error when i run this programme i am using eclipse editor it says scanner statement is not correct.is there anyone can help me to correct this programme
thanks
0 answers
Post by majak maker on May 14, 2012
my question is not about this video. how do you encipher and decipher a text using a key word?
0 answers
Post by Jorge Guerrero on April 2, 2012
Maury, you should have a section on how to setup the user interface. I set mine up and it was a PAIN. I also had to find a workaround for java to run the programs from the command prompt. It was not easy. Also, the NetBeans program does not run individual project files by clicking the huge green button. You need to right click the file in the project window and run just THAT file. Otherwise, NetBeans will run the main class file and may confuse the user, because the default file is written without errors, but outputs nothing. You really need to include this section, please, for the students and newbies. Seems like learning Java is worth a while; I'll see if all my frustration pays off after taking the two courses offered by educator.com. I like to take courses here for intro.
0 answers
Post by Jorge Guerrero on April 2, 2012
Maury, Java is not open-source, even though for now it's free. You should therefore, instruct students on how to setup a developer IDE environment for running Java Script. It won't run on the command prompt either, if you haven't installed the SDK from Oracle's Java site. Neither will NetBeans IDE intall OR run if you don't have the said SDK. The installer takes quite a bit of space on the hard drive, so you should also mention this to students. Once you instruct on what and how to install, THEN you can go ahead and run the scripts. It's not clear for the novice what you're doing or where you're doing it when you're running the example programs.
0 answers
Post by Jialan Wang on March 31, 2012
in 6:05 the program says 500 meters equals 310694.1 miles
what's with that
2 answers
Last reply by: Babatunde Thomas
Mon Mar 10, 2014 6:41 PM
Post by Jacob/Benja Share on January 15, 2012
When I tried to run the first java program in terminal this is what I saw:
Exception in thread "main" java.lang.NoClassDefFoundError: welcome
Caused by: java.lang.ClassNotFoundException: welcome
at java.net.URLClassLoader$1.run(URLClassLoader.java:202)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(URLClassLoader.java:190)
at java.lang.ClassLoader.loadClass(ClassLoader.java:306)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:301)
at java.lang.ClassLoader.loadClass(ClassLoader.java:247).
And this is what I typed in the file "welcome.java":
public class welcome
{
public static void main( string args[] )
{
system.out.println( "Welcome" );
}
}
.
Please tell me what I would do. Thx.
0 answers
Post by Jacob/Benja Share on November 27, 2011
In the Java Script tutorials he said that Java Script and Java have nothing in common but after going through the Java Script this looks very similar.