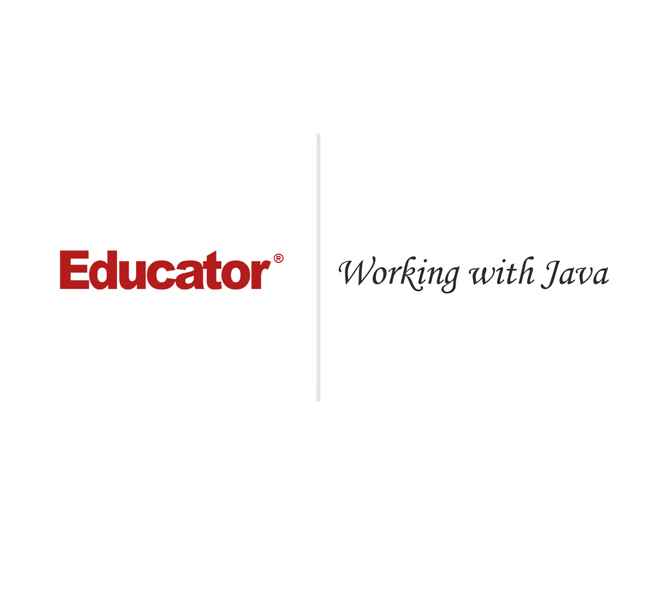

Maury Hillstrom
Working with Java
Slide Duration:Table of Contents
Section 1: Java
Introduction to Java
31m 13s
- Intro0:00
- What is Java?0:12
- Definition of Java0:13
- Writing a Java Program3:48
- Example: Converter IO Program3:49
- Example: Grade Program8:00
- Example: Writing a Java Program12:13
- Starting Point of All Java Code14:00
- 'Public Static Void Main'14:01
- 'Public Static Void Main' Syntax16:07
- Syntax16:08
- String16:27
- args16:41
- How to Add Comments17:13
- How to Add and Use Comments17:14
- Java Statements17:59
- Java Statements and Example18:00
- Example1: Text-printing Program18:44
- Example 2: Text-printing on Multiple Lines20:12
- Example 3: Addition Program that Displays the Sum of Two Numbers21:11
- Example 4: Program that Determines Calculations of Two Numbers24:43
- Example 5: Running Programs Through NetBeans IDE25:50
Working with Java
31m 57s
- Intro0:00
- History of Java0:09
- History of Java0:10
- Requirements of Java2:35
- Free to Download & Install2:36
- System's Path Environment Variable4:40
- Java's Two-Step Process5:48
- Create Source File5:49
- Compile Source File Into Bytecode6:49
- Java Application Creation7:35
- Two Main Ways to Create Java Application7:36
- Other Popular Java-compatible IDE8:08
- Importing Classes8:45
- Importing Classes, Example, and Syntax8:46
- Arithmetic Rules of Precedence10:02
- PEMDAS vs. P(MDR)(AD)(Java)10:03
- Four More Program Examples13:22
- Example: Circle Geometry14:06
- Example: Searching Through a Text String19:50
- Example: Doing a Find & Replace23:35
- Example: Calendar Functions25:45
Classes, Objects, and Methods
29m 34s
- Intro0:00
- Classes, Objects, and Methods0:09
- Class0:10
- Object0:57
- Attributes1:14
- Method1:33
- Arguments1:48
- Instance Variables2:17
- Instance Variables2:18
- Set and Get Methods3:15
- Set and Get Methods3:16
- Primitive Types vs. Reference Types4:11
- Primitive Types4:12
- Reference Type6:56
- Constructors7:22
- Attributes of Constructors7:23
- Example 1: Prints a Table of Squares and Cubes From 0 to 108:26
- Example 2: Bank Account Class with Constructor11:54
- Example 3: Create and Change Account Object14:14
- Example 4: Bank Account Debit Function18:44
- Example 5: Debit Account Balance Program20:08
- Example 6: Hardware Store Inventory & Invoice22:50
- Example 7: Hardware Store Inventory & Invoice Main Class26:05
If Logic
34m 20s
- Intro0:00
- The Mighty 'If'0:08
- If Logic and If Statements0:09
- Conditional Statements in Java1:44
- If1:45
- If…Else2:07
- If…Else If….Else2:33
- Switch3:15
- Pseudocode3:41
- Pseudocode3:42
- Examples4:05
- Compound Assignment Operators6:17
- Compound Assignment Operators and Examples6:18
- Boolean Logical Operators9:02
- && Operator9:03
- | | Operator9:58
- ! Operator11:38
- Example 1: Program that Determines the Larger of Two Numbers13:00
- Example 2: Determine the Largest and Smallest Entry15:55
- Example 3: Determine if a Number is Odd or Even19:24
- Example 4: Determine if Three Values Could Form a Triangle21:44
- Example 5: Run Application for Triangle24:23
- Example 6: Basic If…Else Statement25:11
- Example 7: Basic If…Else If…Else Statement26:52
- Example 8: Catch Possibilities if User Enters Invalid Numbers28:38
- Example 9: Basic Switch Statement31:10
Loops
37m
- Intro0:00
- Types of Loops0:07
- For Loop0:54
- While Loop1:15
- Do…While Loop1:39
- Increment and Decrement Operators2:26
- Operators Overview3:11
- Pre-Increment4:34
- Pre-Decrement5:35
- Post-Increment6:01
- Post-Decrement6:25
- Counters vs. Sentinels7:06
- Counters7:07
- Sentinels7:40
- Break & Continue Statements8:46
- Break8:47
- Continue9:29
- While Loop Syntax10:03
- While Loop Syntax and Example10:04
- For Loop Syntax11:54
- For Loop Syntax and Example11:55
- Example 1: Calculate Commissions for Sales Staff13:55
- Example 2: Program Calculates Average Miles Per Gallon18:56
- Example 3: Find the Smallest of Entered Numbers23:30
- Example 4: Return the Lyrics to 'The 12 Days of Christmas'27:37
- Example 5: Do While Setup31:50
- Example 6: Break Loop Statement Example34:21
- Example 7: Continue Loop Statement Example35:05
Modules
33m 56s
- Intro0:00
- Types of Modules0:09
- Methods & Classes0:10
- Packages0:28
- Static Class Members1:20
- Static Class Members1:21
- Example of Static Class Members1:51
- Queues vs. Stacks3:21
- Queues3:51
- Stacks5:06
- Push and Pop5:42
- Method Overloading6:05
- Method Overloading6:06
- The 'this' Keyword7:13
- 'this' Keyword7:14
- Example7:28
- Composition vs. Inheritance8:05
- Composition and Example8:23
- Inheritance and Example9:33
- Example 1: Roll a Six-diced Die 10,000 Times10:53
- Example 2: Program Calculates Charges for Parking Lot14:47
- Example 3: Test Application for Class Parking20:30
- Example 4: Program that Converts Fahrenheit to Celsius and Vice Versa23:43
- Example 5: Program Simulates Tossing a Coin29:25
Arrays
34m 3s
- Intro0:00
- What is an Array?0:08
- Attributes of Array0:09
- Types of Arrays1:10
- Single-Dimensional1:18
- Two-Dimensional2:01
- How to Declare an Array3:15
- Declaring an Array: Single Dimension3:16
- Declaring an Array: Two Dimension4:12
- The Enhanced 'for' Statement5:02
- 'for' Statement5:03
- Passing Arrays to Methods7:14
- Pass by Value7:34
- Pass by Reference7:54
- Example 1: Simple Array8:24
- Example 2: Program Simulates Rolling Two Six-sided Dice 36,000 Times9:54
- Example 3: Application Roll Two Dice14:49
- Example 4: Program Totals Sales for Sales Staff and Products17:24
- Example 5: Application for Class Sales21:08
- Example 6: Card Class Represents a Playing Card24:43
- Example 7: Deck Class Represents a Deck of Playing Cards26:36
- Example 8: Card Shuffling and Dealing Application30:35
Inheritance
28m 15s
- Intro0:00
- Composition vs. Inheritance0:07
- Composition & Example0:17
- Inheritance & Example1:16
- Composition vs. Inheritance2:04
- Visual Diagram2:05
- Inheritance Class Structure4:36
- Parent Class4:37
- Child Class4:51
- Purpose of Inheritance5:01
- Method Overriding vs. Overloading6:32
- Method Overriding6:39
- Method Overloading7:28
- Inheritance Class Hierarchy8:01
- Inheritance Class Hierarchy8:02
- Protected Members10:27
- Public, Protected, and Private10:28
- Example 1: Basic Inheritance with Constructors12:11
- Example 2: Program Exhibits Both Composition and Inheritance16:03
- Example 3: Class Point Definition21:07
- Example 4: Class Quadrilateral Definition22:15
- Example 5: Class Trapezoid Definition23:09
- Example 6: Class Parallelogram Definition24:05
- Example 7: Class Rectangle Definition24:37
- Example 8: Class Square Definition24:50
- Example 9: Main Application for Shapes Exercise25:42
Polymorphism
36m 53s
- Intro0:00
- What is Polymorphism?0:08
- Definition of Polymorphism0:09
- Polymorphism in Programming1:10
- Superclass1:11
- Subclass2:11
- Variable2:21
- Method2:31
- Polymorphism in Programming3:46
- Superclass3:47
- Subclass4:16
- Variable4:22
- Method4:31
- Abstract Classes vs. Concrete Classes6:31
- Abstract Classes6:32
- Abstract Class Polymorphism Example9:19
- Abstract Superclass9:20
- Concrete Subclasses9:58
- 'Final' Keyword for Superclass Methods13:49
- 'Final' Keyword13:50
- Example14:21
- Example 1: Program to Demonstrate Basic Polymorphism16:25
- Example 2: Program to Demonstrate Polymorphism with Multiple Subclasses18:35
- Example 3: Program Tests Shape Hierarchy25:12
- Example 4: Definition of Class Shape27:17
- Example 5: Definition of Class Two Dimensional Shape28:31
- Example 6: Definition of Class Three Dimensional Shape29:29
- Example 7: Definition of Class Sphere33:45
Exception Handling
22m 45s
- Intro0:00
- What is Exception Handling?0:09
- Definition of Exception Handling0:10
- Why Account for Errors?1:31
- Why Account for Errors?1:32
- Common Types of Errors3:51
- User Caused3:52
- Programmer or System Caused5:31
- Using the 'Try… Catch' Process7:30
- Try7:35
- Catch8:27
- Try… Catch Syntax8:51
- Try… Catch Syntax8:52
- Uncaught Exceptions9:44
- Handling Uncaught Exceptions9:45
- Throw' Statement11:03
- Throw Statement11:04
- Throw Syntax11:22
- Example 1: Program to Catch Divide by Zero Error11:41
- Example 2: Program to Demonstrate Finally Keyword16:18
- Example 3: Superclass and Subclass Exceptions19:20
Loading...
This is a quick preview of the lesson. For full access, please Log In or Sign up.
For more information, please see full course syllabus of Java
For more information, please see full course syllabus of Java
Java Working with Java
Lecture Description
In this lesson our instructor talks about working with Java. First he gives a brief history of Java. Then, he talks about the requirements of Java, Java's two-step process, java application creation, and importing classes. After that, he discusses arithmetic rules of precedence and four more program examples.
Bookmark & Share
Embed
Share this knowledge with your friends!
Copy & Paste this embed code into your website’s HTML
Please ensure that your website editor is in text mode when you paste the code.(In Wordpress, the mode button is on the top right corner.)
×
Since this lesson is not free, only the preview will appear on your website.
- - Allow users to view the embedded video in full-size.
Next Lecture
Previous Lecture
0 answers
Post by idztku on November 7, 2022
How can we run this Java program via Windows? I think you mentioned Bash terminal for Mac, thus i cant figure out how to do for Windows?
0 answers
Post by Scott Yang on July 10, 2019
you are so lucky it wasn't the last day of August. it might say August 32 as tomorrow's date if you did it one day later
0 answers
Post by Scott Yang on July 10, 2019
What if it is the last day of the month? like October 32?
0 answers
Post by Farrukh Awan on October 17, 2018
The video is not working after 18 minutes. Please fix this.
0 answers
Post by Liang Will on March 19, 2017
The video isn't working after 18 minutes. There's nothing wrong with my wifi. Help please.
1 answer
Last reply by: Kitt Parker
Fri Jul 10, 2015 11:59 AM
Post by Elvis Wodi on February 18, 2015
encountered another error message on the replace exercise on this line
String newText =text.Replace('', '-'); // motify the string. saying empty character literal. unclosed character literal. what should i do
1 answer
Last reply by:
Tue Mar 17, 2020 3:44 PM
Post by Elvis Wodi on February 17, 2015
What are %d
and %f
? In the circle example why are we using %d
for diameter and %f
for area and circumference? What is the difference between %d
and %f.Also on the search code i tried following the steps you gave but i encountered and error message on this line (int index =0;)as it says the assigned value is never used.What that mean and how do i go about solving that problem.
1 answer
Last reply by: Jerry Liu
Sun Jun 22, 2014 10:29 PM
Post by Rita Sobiech on September 27, 2013
It isn't going to matter you are coding on a MAC and I have a PC is it?
1 answer
Last reply by: Arshin Jain
Wed Aug 27, 2014 4:09 PM
Post by Tarique Islam on July 16, 2013
I downloaded and setup netbeans (under Windows 8 OS). It works with the simple Hello world stuff..Below is the program:
package myfirstjavaprogram;
/**
*
* @author Owner
*/
public class MyFirstJavaProgram {
int n;
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
System.out.print("Hello world!
");
}
}
But when I typed the "Import.util.Scanner;"
It gives me error! I tried it on the top, as well as within the package statement..
Help
0 answers
Post by joseph kirksey on April 7, 2013
Is Java a good language fer beginners? i've read a little about C++ but the Syntax can be a bit overwhelming for me as a beginner.
1 answer
Last reply by: Phil Wyder
Mon Mar 18, 2013 2:57 PM
Post by Rumman Ahmed on February 17, 2013
What are %d
and %f
? In the circle example why are we using %d
for diameter and %f
for area and circumference? What is the difference between %d
and %f
?
0 answers
Post by Donna Parcel on February 1, 2013
Can you tell me what I'm doing wrong--I downloaded the 4 programs circle, search, find&replace, and calendar and tried to run them in JCreator, NetBeans and they won't run -- I get all kinds of errors.
Thanks Donna
0 answers
Post by anuj singh on January 20, 2013
I am getting error with the dot operator when i try to compile the calendar program on dos what can be the possible reason ?
0 answers
Post by Harlan Reece on December 30, 2012
I tried running the circle program through Netbeans with partial success. My compile was successful, but I did not see a prompt to enter data in the output window. In addition to this problem, I do not see an option to set as Main project option. Is there a preference option where I can set this. When I type enter my project name, it does not simultaneously provide a .Main extension in the Create Main Class field as it does in the video, but rather creates an extension with the exact same name as the project file. What am I doing wrong. Had absolutely no success in running program from DOS prompt. What am I doing wrong?
0 answers
Post by Patricia Edwards on November 11, 2012
in the command line
index = str.indexOf("c");
is the index of str.indexOf the same index as the variable initialized to 0 in the statement above? How does that work?
1 answer
Tue Nov 13, 2012 5:03 PM
Post by Jozsef Kovacs on October 30, 2012
Where can I find java.util.Scanner?
2 answers
Last reply by: Elizabeth Etta
Tue Nov 13, 2012 2:19 PM
Post by Dalbir singh on August 26, 2012
In Circle Geometry example: line 17, 18, 19 why use System.out.printf? why not System.out.println? and how do we know which one to use and when?
3 answers
Thu Nov 1, 2012 2:39 PM
Post by george masih on June 11, 2012
scanner sc = new scanner(system.in);
statement is not working i am using ecllipse editor.any body call tell what is wrong with that?
1 answer
Last reply by: Loammi Pedroza
Tue Apr 16, 2013 8:36 PM
Post by Kwasi Roberts on March 29, 2012
On line 13 on the "Find and Replace" section can you explain tht whole line especially text.replace please?
0 answers
Post by Thomas James Hanrahan on September 29, 2011
Because for some reason the java.util.Calendar; causes the output to be one month behind. I guess that is why you always try to "Break" your code once its complete so you don't run into problems like this.
with +1
Today's date is;9-29-2011
Tomorrow's date will be: 9-30-2011
Without +1
Today's date is;8-29-2011
Tomorrow's date will be: 8-30-2011
2 answers
Last reply by: alina trandafir
Sat May 23, 2015 7:31 PM
Post by Alvaro Lacerda on September 7, 2011
On the 10th line of the calendar program, why do you add "+1" after the "(Calendar.MONTH)"? why that doesn't happen to the other lines "(Calendar.DATE)" and "(Calendar.YEAR)" ?
Thanks,