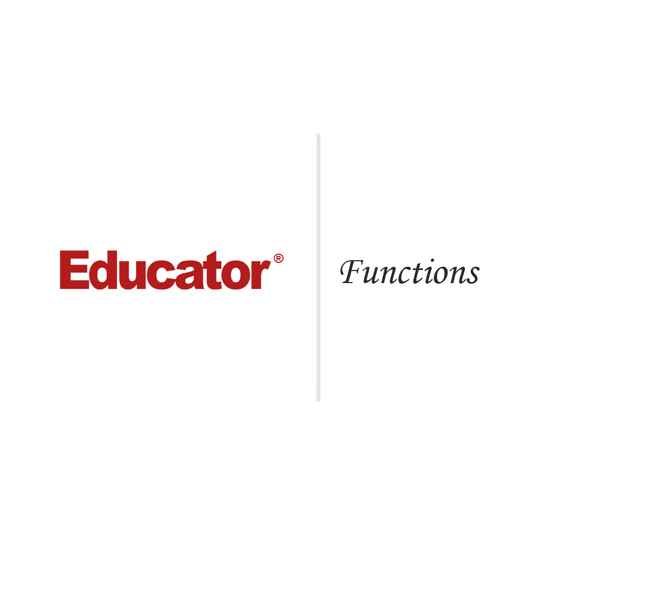
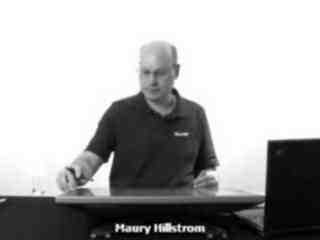
Maury Hillstrom
Functions
Slide Duration:Table of Contents
Section 1: JavaScript
Introduction to JavaScript
22m 43s
- Intro0:00
- What is JavaScript0:07
- Real Life Example 10:28
- Real Life Example 20:56
- Real Life Example 31:31
- Text Editor2:20
- JavaScript vs. Java4:54
- Is JavaScript the Same as Java?4:55
- JavaScript Comment Syntax5:51
- Comment Syntax5:52
- Viewing a JavaScript File6:45
- Browser6:46
- Source Code7:34
- Text Editor7:50
- Example: Browser8:28
- Example: Simple JavaScript File9:13
- Example: JavaScript File10:43
- Example: Font & JavaScript12:25
- Example: Adding Text Color13:37
- Example: Adding a Break Tag16:04
- Example: Adding User Alert Message17:48
- Example: Adding Multi-Line User Alert Message19:02
- Example: Variable & JavaScript20:40
Working With JavaScript
27m 5s
- Intro0:00
- History of JavaScript0:14
- History of JavaScript0:15
- JavaScript vs. Java1:16
- Differences and Similarities Between JavaScript and Java1:17
- Enabling JavaScript4:56
- Running JavaScript in Browsers4:58
- Allowing Active Content: IE6:38
- How to Allow Active Content in Internet Explorer6:40
- Allowing Active Content: Firefox7:36
- How to Allow Active Content in Firefox7:37
- Allowing Active Content: Safari7:59
- How to Allow Active Content in Safari8:00
- Allowing Active Content: Google Chrome8:28
- How to Allow Active Content in Google Chrome8:29
- Pseudocode9:09
- What is Pseudocode?10:20
- Examples: Pseudocode10:45
- Extra Examples12:27
- Basic Math13:01
- Using System Clock and Comparison Operators23:08
If Logic
35m 39s
- Intro0:00
- The Mighty 'if'0:11
- 'If' Statements0:13
- Conditional Statements in JavaScript4:00
- 'If' Statement4:06
- 'If Else' Statement4:36
- 'If Else If Else' Statement5:29
- 'Switch' Statement6:23
- Example 1: If Statement6:47
- Example 2: Two If Statements9:29
- Example 3: Multiple If Statements10:49
- Example 4: If Else Statement14:45
- Example 5: If Else If Else Statement16:32
- Example 6: Employee Pay18:58
- Example 7: Random Number Generator26:36
- Example 8: Switch Statement30:11
Operators
57m 26s
- Intro0:00
- Types of Operators0:09
- Operators Overview0:20
- Arithmetic Operators1:14
- Example: Arithmetic Operators1:15
- Arithmetic Rules of Precedence4:38
- PEMDAS (Algebra)4:59
- P (MDR) (AS) (JavaScript)5:43
- Assignment Operators11:58
- Example: Assignment Operators11:59
- Comparison Operators15:16
- Example: Comparison Operators15:17
- Boolean Logical Operators18:19
- && Operator18:44
- || Operator19:47
- ! Operator22:04
- Special Conditional Operator22:25
- Syntax22:26
- Example23:17
- Increment and Decrement Operators24:03
- Pre-Increment Operator24:38
- Pre-Decrement Operator25:40
- Post-Increment Operator26:19
- Post-Decrement Operator27:07
- Example 1: Modulus Operator27:53
- Example 2: Assignment Operator32:14
- Example 3: Characters and Data Types37:57
- Example 4: Characters and Data Types43:17
- Example 5: Boolean Logical Operators44:56
- Example 6: 'or' Logic46:43
- Example 7: Conditional Operators49:39
- Example 8: Increment and Decrement Operators52:18
Loops
23m 42s
- Intro0:00
- Types of Loops0:05
- For Loop0:54
- While Loop1:19
- Do…While Loop2:03
- For…in Loop2:48
- Main Purpose of Loops3:57
- Example 1: For Loop4:21
- Example 2: For Loop with Total7:00
- Example 3: For Loop in HTML10:31
- Example 4: Reverse For Loop12:02
- Example 5: While Loop14:08
- Example 6: Do While Loop15:50
- Example 7: Break Loop17:06
- Example 8: Late Break Loop18:42
- Example 9: Continue Break Loop20:05
- Example 10: For…in Loop21:28
Functions
31m 55s
- Intro0:00
- Q & A0:08
- Questions and Answers0:14
- Two Main Types of Functions1:11
- JavaScript Library Functions1:26
- Programmer-Defined Functions1:38
- Library Functions1:55
- JavaScript Library Functions1:56
- Examples2:23
- Programmer-Defined Functions3:01
- Procedure3:02
- Programmer-Defined Functions4:05
- Function Definition Syntax4:10
- Example 1: Delayed Function5:46
- Example 2: Squared Numbers8:15
- Example 3: Exponents12:08
- Example 4: One Die16:13
- Example 5: Area of Circle22:09
- Example 6: Area of Circle With Exponent25:53
- Example 7: Temperature Converter27:21
Events
24m 24s
- Intro0:00
- Object-Oriented, Event-Driven Programming0:08
- OBJECT + EVENT = PROGRAM0:10
- Examples of Objects1:49
- Windows/OS Objects1:52
- Examples of Objects2:48
- JavaScript Objects2:49
- Examples of Events3:19
- Mouse Clicks3:27
- Mouse-Overs3:39
- Keystrokes4:17
- Page Loads4:38
- Other User Inputs5:07
- Examples of Programs5:34
- Examples5:42
- Summary6:41
- OBJECT + EVENT = PROGRAM6:42
- Example 1: Graphic Object7:20
- Example 2: Mouse Over Events10:36
- Example 3: X/Y Screen Pixel Coordinates14:22
- Example 4: Keystroke Detector17:21
- Example 5: Event Bubbling20:51
Error Handling
21m 25s
- Intro0:00
- Using Try..Catch to Detect Errors0:09
- What is Handling Errors?0:23
- Try..Catch Syntax1:22
- Throwing Exceptions2:13
- Throw Statement Syntax2:15
- Special Text Characters2:57
- Special Text Characters2:58
- Example 1: Try...Catch4:51
- Example 2: Try…Catch Correction8:32
- Example 3: Try…Catch Redirection10:53
- Example 4: Try Catch Throw12:14
- Example 5: Throwing Errors18:24
- Example 6: Special Characters Error19:51
- Example 7: Special Text Characters20:38
Objects
26m 15s
- Intro0:00
- Definitions0:08
- Objects0:28
- Methods0:44
- Properties1:01
- Example1:22
- String Object1:26
- Test Object2:15
- Common JavaScript Objects3:10
- Array Object3:25
- Boolean Object3:44
- Date Object3:52
- Form Object4:09
- Math Object4:48
- Number object4:58
- String Object5:27
- Window Object5:32
- Document Object5:48
- Regular Expression Objects5:55
- Regular Expression Syntax5:57
- Example 1: Object Method7:04
- Example 2: Object Property8:41
- Example 3: String Object Styles9:09
- Example 4: String Match Method11:00
- Example 5: String Replace Method12:45
- Example 6: Web Page Clock14:05
- Example 7: Math Object Absolute Value15:25
- Example 8: Minimum and Maximum Values17:05
- Example 9: Form Objects18:47
- Example 10: Regular Expressions23:22
Arrays
18m 19s
- Intro0:00
- What is an Array?0:09
- Defining an Array0:10
- Declaring an Array1:30
- Declaring an Array1:31
- Regular Array Syntax1:47
- Regular Array Syntax1:48
- Condensed Array Syntax2:35
- Condensed Array Syntax2:36
- Literal Array Syntax3:08
- Literal Array Syntax3:09
- Push and Pop3:30
- Push and Pop Methods3:32
- Example 1: Array Creation4:18
- Example 2: Array Element Display6:30
- Example 3: Array Pop Method7:39
- Example 4: Array Push Method9:12
- Example 5: Array Reverse Method10:22
- Example 6: Array Shift Method11:27
- Example 7: Array Sort Method12:53
- Example 8: Array Slice Method13:53
- Example 9: Array Splice Method16:42
Loading...
This is a quick preview of the lesson. For full access, please Log In or Sign up.
For more information, please see full course syllabus of JavaScript
For more information, please see full course syllabus of JavaScript
JavaScript Functions
Lecture Description
In this lesson our instructor talks about functions. He starts with questions and answers. Then he talks about two main types of functions such as JavaScript library functions and programmer-defined functions. He discusses library functions and shows some examples. He talks about procedure and function definition syntax for programmer-defined functions. Seven complete example videos round up this lesson.
Bookmark & Share
Embed
Share this knowledge with your friends!
Copy & Paste this embed code into your website’s HTML
Please ensure that your website editor is in text mode when you paste the code.(In Wordpress, the mode button is on the top right corner.)
×
Since this lesson is not free, only the preview will appear on your website.
- - Allow users to view the embedded video in full-size.
Next Lecture
Previous Lecture
1 answer
Last reply by: Hong Yang
Wed Sep 16, 2020 4:23 PM
Post by DJ Sai on August 9, 2018
How is no one realizing the square of number "x" should be x*x, not x*x*x?
0 answers
Post by Ebssa Tesema on July 1, 2015
Hei
Why do we need the function square (y)? how do we manage to exchange the values between x and y.
I see we have var x in the for loop where it is going to increment to 10; i also understand that we havn't given the square (x) function it is proper calculation instead we used function square (y);
and we are getting the result!!! ok but what does it mean to pass data?
can you please answer this question??
1 answer
Sun Feb 2, 2014 3:45 PM
Post by lae loe on January 28, 2014
Hello Maurry... I try this code and java script said missing radix parameter..
these are the two codes that show mssing radix parameter and the exponent didn't work..
original = parseInt(form.original.value);
exponent = parseInt(form.exponent.value);
function getExponent(){
var form = document.getElementById("exponentForm");
var original;
var exponent;
original = parseInt(form.original.value);
exponent = parseInt(form.exponent.value);
form.result.value = numP(original,exponent);
}
function numP(original, exponent)
{
var result = 1;
for(var i = 0; i <exponent; ++i);
result *= original;
return result;
}
1 answer
Sun Feb 2, 2014 3:43 PM
Post by lae loe on January 28, 2014
Hello Maurry... I try this code and java script said missing radix parameter..
these are the two codes that show mssing radix parameter and the exponent didn't work..
original = parseInt(form.original.value);
exponent = parseInt(form.exponent.value);
function getExponent(){
var form = document.getElementById("exponentForm");
var original;
var exponent;
original = parseInt(form.original.value);
exponent = parseInt(form.exponent.value);
form.result.value = numP(original,exponent);
}
function numP(original, exponent)
{
var result = 1;
for(var i = 0; i <exponent; ++i);
result *= original;
return result;
}
1 answer
Tue Nov 13, 2012 4:53 PM
Post by Clarence Eppinger on November 12, 2012
where and how does the value of "y" get set tto the current value of "x"?
0 answers
Post by Grady Ela on June 4, 2012
I am curious as to why the variable "x" is used at the top in the "for" statement but the variable "y" is used in the function. I noticed that they are interchangeable if you change all of the variables but I would like some clarification.
Thanks!
0 answers
Post by Jorge Guerrero on March 31, 2012
Very illustrative. I'm getting closer to deploying web applications the way I do in MS Access.
What I've noticed is that commercial languages such as VBA and VB are similar to echother, but open source langauges such as JavaScript and PHP are more similar to eachother.
Nevertheless, MS Access is a good start to manage desktop databases and from there mimic web applications for the same power.
Both are useful.