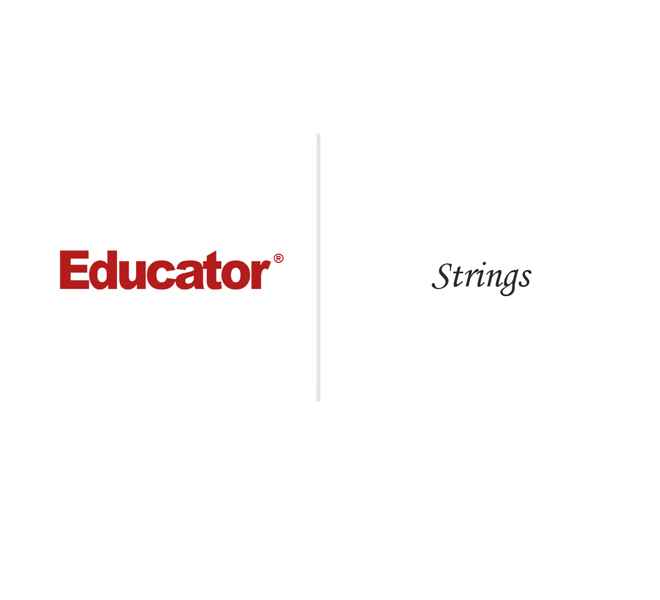
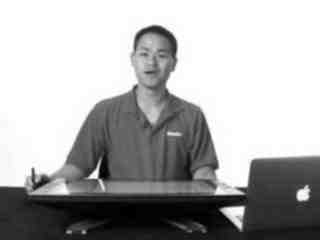
Justin Mui
Strings
Slide Duration:Table of Contents
Section 1: Introduction to Ruby
Setting Up Your Environment
22m 8s
- Intro0:00
- Installing Ruby0:06
- Ruby-lan.org0:07
- Three Ways of Installing Ruby2:26
- Compiling Ruby-Source Code3:02
- Third Party Tools3:28
- Other Implementations of Ruby4:48
- Windows Installation5:21
- RubyInstaller.org5:22
- Mac OSX and Linux Installation6:13
- Mac OSX and Linux Installation6:14
- Setting Up Debian/Linux6:42
- Setting Up Debian/Linux6:43
- Installing HomeBrew6:56
- HomeBrew for MAC OSX6:57
- HomeBrew Wiki9:44
- Installing HomeBrew10:02
- Setting Up Mac OSX11:46
- HomeBrew, RVM, OSX-GCC Installer, and Install Ruby 1.9.311:47
- Ruby Version Manager (RVM)12:11
- Ruby Version Manager (RVM) Overview12:12
- Installing Ruby Version Manager (RVM): http://rvm.io12:35
- Install RVM with Ruby14:20
- Install RVM with Ruby14:21
- Install OSX-GCC-Installer16:18
- Download and Install Package for Your OSX16:19
- Install Ruby 1.9.317:28
- Install Ruby 1.9.317:29
- Test It Out!18:09
- rvm-help & ruby-v18:10
- Example: rvm gemset create educator18:52
- Set It As Default!20:47
- rvm Use 1.9.3@educator--default20:48
Intro to Ruby
22m 20s
- Intro0:00
- What is Ruby?0:06
- What is Ruby?0:07
- Ruby Standard Library0:52
- Who Created Ruby?1:22
- Yukihiro Matsumoto1:23
- History2:45
- The Name 'Ruby'2:46
- Ruby v0.953:10
- Ruby v1.03:56
- English Language Mailing List Rubytalk4:08
- ruby-forum.com & the Mailing Lists4:27
- Ruby In The West9:51
- Ruby on Rails10:39
- The Pragmatic Programmer's Guide to Ruby11:30
- rubyonrails.org13:34
- Current Ruby14:42
- Ruby 1.8.7, Ruby 1.9.3, and Ruby 2.014:43
- Why Programmers Enjoy Ruby?15:40
- Why Programmers Enjoy Ruby?15:41
- Ruby Is An Interpreted Language16:21
- Ruby Is An Interpreted Language16:22
- What Is It Used For?16:50
- What Is It Used For?16:51
- Ruby is Object-Oriented18:17
- Example: 5.class18:18
- Example: 0.0.class18:54
- Example: true.class19:03
- Example: nil.class19:12
- Object Class19:19
- BasicObject19:20
- Example19:52
- Superclass20:50
- Fixnum → Integer → Numeric → Object21:32
Basic Tools for Using Ruby
27m 44s
- Intro0:00
- Interactive Ruby0:08
- irb: Interactive Command-Line Environment0:09
- Example0:49
- irb-v0:50
- irb-executes terminal1:02
- 1.9.3-p125 > 'hi'1:09
- Live Demonstration1:31
- Why Use Interactive Ruby?2:21
- Why Use Interactive Ruby?2:22
- RDoc3:05
- RDoc3:06
- Ruby Core Documentation3:32
- Ruby Core Documentation: Example5:30
- Ruby Core Documentation: Markup6:12
- Ruby Core Documentation: Headings7:44
- Coding Example: RDoc9:30
- Why Use RDoc?13:02
- Learning Core Ruby Functions13:03
- Generating RDoc15:31
- rdoc-help # usage15:32
- Ruby Interpreter15:57
- ruby -- help15:58
- ruby [switches] [-] program [arguments]16:16
- Example: How to Run a Ruby Script16:28
- Rake18:38
- Rake Overview18:39
- Ruby Core Documentation: Rake19:46
- Coding Example: Rake23:14
- Why Was It Created?24:30
- Why Was It Created?24:31
- Lesson Summary25:13
- Lesson Summary25:14
- IDE/script Editors: MacVIM26:24
Ruby Specifics
20m 45s
- Intro0:00
- Ruby Specifics0:06
- Comments0:51
- Hashtags1:00
- Example1:23
- Multi-Line Comment2:04
- Example3:10
- RDoc Comments4:02
- When do you generate an Rdoc?4:10
- Headings and subheadings4:24
- Examples4:48
- Generating an Rdoc - example4:50
- Common Code Conventions6:28
- For every tab use two spaces indentation7:38
- Never use tabs7:42
- Common Code Conventions (Cont.)8:18
- Camel case8:20
- Snake case9:18
- Identifiers9:44
- Constants begin with CAP letter10:00
- Examples10:10
- Identifiers with Different Scoping10:26
- Global10:34
- Instance Variable10:40
- Class Variable10:46
- Examples10:56
- Reserved Keywords12:22
- Do not use reserved keywords in code12:25
- Parentheses are Sometimes Optional13:04
- Functions do not require parentheses13:16
- When in doubt, use parentheses13:54
- Examples14:10
- Newlines Are Statement Terminators14:20
- Examples15:10
- Continuation with a Period16:20
- Period means continue to next line16:46
- Multiple Statements Allowed on a Single Line17:38
- Try not to use semi-colons17:58
- Code Blocks18:20
- Use code blocks for one liners18:28
- Examples18:40
- Recommended for multiple lines20:16
Ruby Data Types (Part 1)
29m 37s
- Intro0:00
- Overview0:10
- Ruby Data Types0:10
- Numbers0:16
- Strings0:18
- Symbols0:24
- Numbers0:30
- Numeric0:44
- Float0:50
- Complex0:56
- BigDecimal0:58
- Rational1:00
- Integer (most popular) - Fixnum and Bignum1:06
- Fixnum stores 31 bits1:18
- Bignum stores larger bits1:24
- All number objects are instances of Numeric1:28
- Integer Literals2:28
- Represent whole-numbers2:40
- Examples - Different bases2:42
- Binary3:04
- Octal3:30
- Hexadecimal3:44
- Examples4:06
- Floating Point Literals4:45
- Examples4:58
- e-value can be capital or lowercase5:30
- Example5:44
- Strings6:16
- Mutable objects6:18
- Used for inserting and deleting text, searching, and replacing6:26
- String Rdoc6:46
- Definition7:00
- String Literals8:20
- Single-Quoted8:28
- Double-Quoted (most used)8:50
- Example9:32
- Escape Sequences11:10
- Newline11:16
- Tab11:22
- Double quote11:28
- Blackslash11:36
- Interpolation11:50
- Sprintf13:48
- Unicode Escaping14:38
- Example15:50
- Delimiters16:18
- Here Documents17:18
- Example17:25
- String Operators19:58
- Concatenation20:03
- Appending20:40
- String Equality21:04
- Example21:24
- Substrings22:00
- Range object (inclusive)22:22
- String Encoding24:52
- Differences between Ruby 1.8 and 1.924:56
- Symbols26:02
- Definitions26:04
- Examples26:46
- When to use symbols26:54
- Symbols and Strings27:42
- Symbols Rdoc28:22
Ruby Gems
25m 50s
- Intro0:00
- RubyGems0:08
- What are RubyGems?0:24
- RubyGems.org0:44
- How RubyGems are used2:06
- Java's jar utility2:50
- Unix/Linux's tar utility3:06
- What is a Gem?3:16
- Definition of Gem3:20
- Version3:34
- Date3:44
- Author3:50
- Description5:58
- What Are the Uses?4:18
- Uses for Gems4:22
- Installation5:06
- How to install RubyGems5:30
- Updating to the Latest Ruby Gems5:54
- Testing6:22
- Example6:34
- Installing Rake7:24
- Example7:46
- Verifying9:22
- Example9:56
- Structure10:56
- gem.gemspec11:30
- Specification13:40
- What is in the gem?13:42
- Who made it?13:50
- Update gem version13:58
- Example14:10
- Create Our First Gem17:20
- Steps involved17:28
- RubyGems Guides17:36
- Example20:02
- Steps Review18:56
- Create Our First Gem (Cont.)23:08
- Building the gem19:38
- Example20:00
- Installing the gem22:32
- Run it22:52
- Publish it23:04
- Get Some Gems!25:06
- rake25:14
- rails25:19
- fastercsv25:25
- koala25:37
Ruby Data Types (Part 2)
40m 24s
- Intro0:00
- Ruby Data Types0:15
- Boolean0:21
- Arrays0:27
- Hashes0:33
- Range0:37
- Boolean Types0:42
- TrueClass0:56
- FalseClass1:12
- NilClass1:18
- TrueClass Examples2:48
- FalseClass Examples3:22
- Arrays4:16
- Ordered collection of objects4:22
- Can hold different objects4:32
- Starts at index 04:50
- Array of Strings5:50
- Example5:52
- Arrays (Cont.)6:20
- Can be created using literals6:22
- Can be created using constructors6:54
- Position and indexed value8:04
- Negative Indexed Values8:56
- Shift and Unshift10:18
- Push and Pop11:38
- .delete method12:38
- Addition and Subtraction13:32
- Union and Intersection14:48
- Insert15:52
- Iteration16:52
- Arrays Rdoc17:48
- Hashes22:08
- Maps and Associative Arrays22:44
- Created using the constructor22:56
- Created using a hash literal24:02
- Stored in a hash table25:26
- Example25:50
- Accessing Key-Values27:46
- Deletion29:48
- Iteration31:04
- Hashes Rdoc32:04
- Ranges36:40
- Two dots are inclusive36:57
- Three dots are exclusive37:16
- Example37:50
- Ranges Rdoc38:24
Objects
1h 5m 46s
- Intro0:00
- Objects0:10
- Object References1:36
- Ruby Core2:16
- Example4:30
- Creating New Objects6:00
- New Method6:08
- Initialize Method6:31
- Example7:18
- Garbage Collection9:54
- Global values always reachable10:25
- Object Identity11:08
- Every object has an object identifier11:20
- Object identifier is constant and unique11:30
- Example11:54
- Object Class12:58
- Class method13:10
- Superclass method13:28
- Object Testing14:46
- is_a?15:49
- respond_to?16:26
- String and Regexp18:10
- Comparing two object instances20:06
- Example23:30
- Object Equality25:48
- Comparing objects25:54
- equal?25:58
- Popular way to test for equality27:16
- Opposite way to test for equality27:25
- Arrays28:30
- Hash29:42
- Case equality operator30:47
- Class tests31:16
- Range tests31:48
- Symbol tests32:32
- Object Conversion33:14
- Explicit conversion33:54
- Implicit conversion35:00
- Example36:12
- Object Conversion: Kernel Module38:22
- Array38:38
- Float39:26
- Integer39:58
- String40:10
- Example40:34
- Object Conversion: Coerce42:00
- Used for mixed type numeric operations42:08
- Example43:40
- Object Conversion: Boolean47:42
- Every object has a boolean value47:44
- Example48:54
- Object Copying50:52
- dup50:58
- clone51:03
- Example51:42
- Object Freezing57:36
- Object Marshaling58:38
- Save state59:04
- Load state59:27
- Example59:32
- Tainted Objects1:01:50
- taint1:02:08
- farm field1:02:12
- Untrusted Objects1:04:06
- trust1:04:24
- untrust1:04:34
- untrusted?1:04:42
Loops
38m 54s
- Intro0:00
- Loops0:12
- while and until0:48
- for and in0:54
- iterators1:04
- enumerable in objects1:06
- While-loop1:14
- Will keep going is condition is true1:18
- Until-loop2:58
- Will keep going until condition becomes true3:06
- Single Expression Loops4:20
- Compact form4:30
- Expressed as a modifier4:42
- Do-While Loop5:52
- Executes body first6:06
- Do-Until Loop7:54
- Similar to do-while loop7:58
- Using Break Inside Loops8:54
- break8:58
- For-In Loop11:56
- for-loop12:06
- var12:34
- collection12:54
- body13:00
- Examples13:08
- Examples (Cont.)15:54
- Nested loops16:40
- Numeric Iterators18:32
- upto18:40
- downto18:42
- times18:48
- Examples20:28
- External Iterators21:00
- Enumerator class21:04
- Rdoc21:16
- Enumerables in Objects24:35
- Enumerable is a mix-in24:41
- RDoc25:24
- Commonly Used Enumerables in Objects27:01
- Array27:19
- Hash27:51
- Range28:47
- Examples29:29
- Enumerables in Objects (Cont.)31:13
- File Processing31:15
- Example31:45
- Enumerables in Objects (Cont.)33:07
- collect33:23
- select34:11
- reject34:59
- inject35:29
Strings
28m 30s
- Intro0:00
- Strings0:08
- Why do you want to get familiar with strings?1:00
- String Creation1:16
- new1:28
- empty?1:50
- length or size2:10
- Example3:12
- String Manipulation4:40
- slice4:56
- square brackets [ ]5:02
- token5:40
- [fixnum]6:52
- offset and length8:40
- chaining12:42
- String Insertion12:56
- insert12:58
- positive or negative index13:46
- String Updates15:24
- [token]15:36
- Examples16:40
- chop or chop!17:54
- chomp!18:56
- gsub20:28
- String Deletion21:38
- delete21:38
- String Reversal22:46
- reverse22:52
- String Manipulation23:16
- split(pattern=$, limit)23:22
- pattern24:10
- limit24:15
- upcase or upcase!25:28
- downcase or downcase!26:02
- swapcase26:24
- Incrementing Strings27:26
- next or next!27:32
- Check Out the Other Lessons28:00
- Ruby Data Types Part 128:12
- Regular Expressions28:18
Regular Expressions
33m 27s
- Intro0:00
- Regular Expressions0:10
- How to create a regular expression0:48
- What goes inside1:36
- Metacharacters3:10
- Bracket expressions3:14
- Quantifiers3:18
- Anchors3:20
- Metacharacters3:30
- word and non-word characters4:04
- digit and non-digit characters4:44
- hexdigit and non-hexdigit characters4:56
- whitespace and non-whitespace characters5:08
- Examples5:24
- POSIX Bracket Expressions7:48
- Non-POSIX Bracket Expressions9:48
- Bracket Expression Examples10:58
- Quantifiers12:34
- Examples13:30
- Character Properties17:24
- Similar to POSIX bracket classes18:22
- More Character Properties18:48
- Examples19:32
- Anchors20:08
- Examples21:14
- Regular Expression Matching: Regexp Object22:40
- match22:51
- Regular Expression Matching: String Object24:14
- match24:26
- Regular Expression Modifier Characters25:14
- pat25:38
- Example26:42
- Regular Expression Modifier Objects27:14
- Example28:38
- Regexp Rdoc30:40
Arrays
14m 35s
- Intro0:00
- Arrays0:12
- Creating an Array with a Block0:50
- Alternative Ways to Create an Array3:52
- Checking the Class5:14
- Iterate through the array5:26
- Call the class method5:28
- Array Shortcuts6:38
- at(index)6:44
- delete_at(index)7:28
- first(n)8:28
- last(n)9:28
- Removing Duplicates9:58
- uniq or uniq!10:04
- Sorting the Array10:48
- sort or sort!10:54
- Getting the Index11:35
- index11:56
- rindex12:38
- Multidimensional Arrays12:56
- flatten13:33
- Check Out the Earlier Lesson14:16
- Ruby Data Types Part 214:26
Hashes
27m 48s
- Intro0:00
- Hashes0:12
- Creating Hashes1:18
- Setting a Default Value2:24
- Accessing Hashes4:16
- Accessible by keys or by values4:28
- Keys must be unique4:36
- Creating Hashes5:16
- Comma-separated list5:42
- Hash rocket8:28
- Examples10:16
- Iterating Keys and Values11:43
- each_key12:04
- each_value14:04
- Merging Hashes16:10
- merge(other_hash)16:20
- Sorting Hashes18:46
- Replacing Hashes20:57
- replace(other_hash)21:18
- Converting Hashes to Other Classes22:04
- to_a22:22
- to_s23:22
- Example24:34
- Check Out the Earlier Lesson27:22
- Ruby Data Types Part 227:32
Math Operations, Part 1
28m 47s
- Intro0:00
- Math Objects0:12
- Numeric0:26
- Integer0:38
- Float1:02
- Fixnum1:14
- Bignum1:56
- Rational2:04
- Math2:24
- Math Operations2:36
- Example3:14
- div(numeric)4:54
- divmod(numeric)6:30
- modulo(numeric)7:23
- quo(numeric)8:18
- remainder(numeric)9:35
- Operation Precedence 1 of 310:35
- Operation Precedence 2 of 313:18
- Operation Precedence 3 of 314:28
- Abbreviated Math Operations14:54
- Move the operator in front of the equal sign15:52
- Numbers16:36
- Numeric Class17:06
- Numeric Methods18:41
- ceil18:52
- floor19:32
- round19:50
- Example with Numbers20:20
- Numeric Methods (Cont.)22:20
- truncate22:28
- num.step(limit, step)23:02
- Numeric Rdoc25:26
Math Operations, Part 2
28m 51s
- Intro0:00
- Math Operations0:12
- Math Module0:24
- Rational Numbers0:44
- Complex Numbers0:52
- Prime Numbers0:58
- Matrices1:06
- Math Module1:12
- PI and E1:32
- Math Module Methods2:47
- atan2(x,y)2:56
- cos(x)3:14
- exp(x)3:44
- Examples4:38
- log(x)5:44
- log(num, base)6:34
- log10(x)7:04
- sin(x)7:34
- sqrt(x)7:52
- tan(x)8:06
- Math Functions: Part 1 of 38:12
- Math Functions: Part 2 of 39:32
- Math Functions: Part 3 of 310:19
- Math Module Rdoc11:25
- Rational Numbers13:23
- How to use14:06
- Example15:02
- Mathematical Ruby Scripts (Mathn)16:25
- Example17:28
- Complex Numbers18:26
- polar18:56
- rect19:10
- Complex Number Examples19:18
- Prime Numbers20:14
- each(ubound=nil)20:44
- prime?21:22
- Example21:58
- Matrices23:15
- build(row_size, column_size=row_size)23:44
- Example24:44
- Matrix Rdoc24:58
Dates and Times
26m 1s
- Intro0:00
- Dates and Times0:12
- Time Class0:38
- Methods of the Time Class1:43
- now1:44
- at(time)2:10
- Epoch & Unix Timestamp Conversion Tools3:19
- Components of a Time5:07
- Convert Time to an Array5:54
- to_a6:08
- Creating a New Time6:48
- Time.local7:08
- Year is required7:22
- Time.utc8:12
- What should be specified9:30
- More Methods of the Time Class10:16
- strftime(string)11:26
- RDoc12:50
- Date Library16:46
- Initializing a New Date17:08
- Parsing Dates18:28
- parse(string)18:42
- Today's Date19:19
- Date.today19:22
- Tomorrow's Date20:22
- Next20:28
- Next week21:22
- Count Down21:26
- Count Up22:37
- Components of a Date23:20
- Converting to Datetime23:48
- to_datetime24:00
- Initializing a Datetime24:24
- Converting to Time25:23
- self.to_time25:32
Methods: Part 1
31m 24s
- Intro0:00
- What is a Method?0:12
- Basic Method0:58
- Return Value4:37
- return4:46
- Factorial Example6:18
- Example8:46
- Return Two Values10:06
- Set the return keyword10:14
- Collected and returned as an array10:28
- Undefining Methods11:22
- undef method_to_undefine11:44
- Example12:32
- Method Names13:02
- Begin with lowercase letter13:16
- Separate longer words with underscores13:26
- Can end with equal sign, question mark, or exclamation point14:03
- Equal sign14:26
- Method Names with Question Mark14:44
- empty?15:24
- Method Names with Exclamation Point16:01
- mutators16:12
- ! means use with caution16:46
- Method Aliases18:05
- alias new_method existing_method18:42
- Operator Methods20:00
- Operators20:02
- Array Operators20:10
- Unary Operators20:32
- Binary Operators20:40
- Example21:28
- Methods and Parentheses25:00
- Optional in most cases25:20
- Required in other cases27:13
- Methods and Blocks27:54
- Associated with blocks28:18
- block_given?28:26
- yield28:36
- Example29:24
Methods: Part 2
20m 11s
- Intro0:00
- Methods with the Unary Ampersand Operator0:14
- &0:34
- Block to a Proc0:56
- Example2:02
- Proc object3:58
- Example5:04
- Methods with Default Values5:54
- Example7:12
- Methods with variable-Length Arguments8:05
- How to create it8:36
- Example11:06
- Using Hashes with Arguments13:02
- Multiple arguments13:08
- Solution13:30
- Example14:56
- Rdoc18:12
Classes: Part I
26m 51s
- Intro0:00
- Classes0:10
- Definition of a class0:14
- Class represents a container0:32
- Can be reused0:46
- Creating our First Class1:00
- Keyword class will create new class1:06
- Name must begin with capital letter1:30
- Instantiating Our First Class2:46
- New will create a new instance of class2:58
- Initializing Values3:58
- Definition of def4:14
- Instance method5:08
- Example7:02
- Defining the to_s Method8:24
- Creating a string representation class8:34
- Example10:54
- Self in the Class12:16
- Definition of self12:26
- Example13:54
- Accessor Methods15:52
- getter methods16:22
- Example17:00
- Setter Methods18:00
- Mutator methods18:02
- Example19:46
- Automating Getter and Setter Methods21:10
- Defined in the module class21:30
- attr_reader21:54
- attr_writer22:48
- attr creates getter and setter methods23:50
- Example24:28
- Notes on Ruby's Accessor Methods25:32
Classes: Part II
26m 42s
- Intro0:00
- Defining Operators0:10
- You can define arithmetic operators0:32
- Unary Operators0:46
- Let's define operators in our class!0:56
- Example2:52
- Class Methods6:24
- Examples6:56
- Opening Up the Class9:38
- Adds an additional method9:54
- Examples11:04
- Array and Hash Access Method15:40
- Use square brackets16:02
- Define your own has access method16:08
- Example16:56
- Enumerating The Values18:40
- Define the each iterator18:40
- Testing for Equality19:36
- Class Triplex19:50
- Examples20:54
- Constants25:00
- Usually defined at the top of class25:24
Classes: Part III
53m 36s
- Intro0:00
- Class Variables0:14
- Example2:16
- Ruby Glass Jar Example8:50
- Class Instance Variables10:20
- Instance variables of class objects10:46
- Advantage of class instance variables11:18
- Examples11:30
- Method Visibility16:16
- Three types of method visibility16:26
- Public methods17:34
- Private methods17:38
- Protected methods18:04
- Invoking Method Visibility19:21
- Public , Protected, and Private Visibility19:22
- Invoking Method Visibility With Arguments21:39
- Example: Invoking Method Visibility22:12
- Class Visibility23:31
- Instance and Class Variables are Private23:32
- Constants are Public24:00
- Makes Existing Class Methods Private24:27
- Makes Existing Class Methods Public25:08
- Example: Class Visibility and class GlassJar25:43
- Subclassing27:08
- Subclassing: Subclass and Superclass27:09
- Example: Subclassing29:43
- Inheritance30:05
- Inheritance30:06
- Example: Inheritance31:25
- Subclassing and Inheritance31:34
- Descendants31:41
- Ancestors31:56
- More On Descendants and Ancestors32:08
- Extending a Class33:27
- Extending a Class33:28
- Coding Example: Extending a Class34:24
- Overriding a Method36:41
- Overriding a Method36:42
- Coding Example: Overriding a Method37:18
- Modifying Methods with Chaining38:52
- Modifying Methods with Chaining38:53
- Super39:25
- Coding Example: Modifying Methods with Chaining39:51
- The Singleton Pattern44:52
- Introduction to The Singleton Pattern44:53
- Setting Up Singleton45:28
- The Instance Method45:58
- Rdoc for Singleton: Usage46:23
- Rdoc for Singleton: Implementation47:45
- Coding Example: Singleton49:38
Modules
24m 19s
- Intro0:00
- Modules0:04
- What is Modules?0:05
- Modules Examples0:40
- Modules: Mix-Ins3:31
- What is a Mix-in?3:32
- Modules: Namespace4:07
- What is a Namespace?4:08
- Why Use a Namespace?5:13
- Example of a Namespace Module5:59
- Example of Mixing in The Module Into the Global Scope6:00
- Modules: Creation7:04
- How to Create a New Module?7:05
- Modules: Usage8:19
- How to Use It?8:20
- class Poker & class Bridge9:13
- Creating Our Module as a Mix-In9:41
- Example of a Module Using Instance Methods9:42
- Coding Example10:20
- Creating Our Module as a Namespace12:11
- Implement Class Methods for the Module12:12
- Coding Example14:56
- Loading Our Module19:46
- Loading Our Module Overview19:47
- Require & Load20:15
- Coding Example: Loading Module20:48
- Lesson Summary23:36
Loading...
This is a quick preview of the lesson. For full access, please Log In or Sign up.
For more information, please see full course syllabus of Introduction to Ruby
For more information, please see full course syllabus of Introduction to Ruby
Introduction to Ruby Strings
Lecture Description
In this lesson our instructor talks about string. First he discusses string creation, string manipulation, string insertion, and string updates. Then he talks about string deletion, string reversal, string manipulation, incrementing strings, and other lessons.
Bookmark & Share
Embed
Share this knowledge with your friends!
Copy & Paste this embed code into your website’s HTML
Please ensure that your website editor is in text mode when you paste the code.(In Wordpress, the mode button is on the top right corner.)
×
Since this lesson is not free, only the preview will appear on your website.
- - Allow users to view the embedded video in full-size.
Next Lecture
Previous Lecture
Start Learning Now
Our free lessons will get you started (Adobe Flash® required).
Sign up for Educator.comGet immediate access to our entire library.
Membership Overview