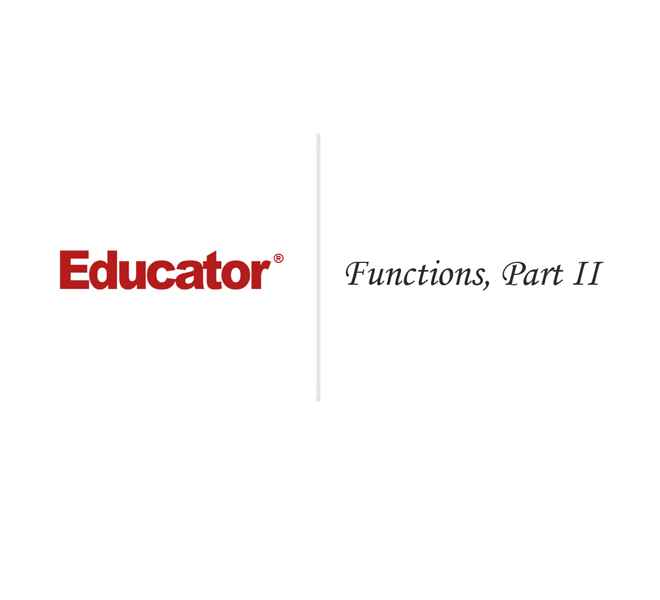
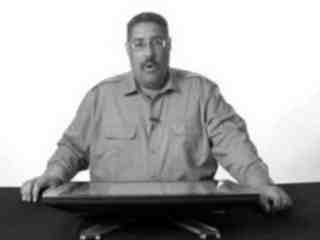
Alvin Sylvain
Functions, Part 2
Slide Duration:Table of Contents
Section 1: Introduction to C++
Introduction
26m 51s
- Intro0:00
- Overview0:11
- What You'll Learn0:51
- Evaluate & Write Some Real C++ Code3:22
- Learn Some 'Best Practices'3:43
- C++ in the Wild4:01
- Examples7:11
- Declare an Integer7:20
- Call Functions7:41
- Examples10:20
- Factorial10:36
- Loop12:20
- Examples13:20
- If Greater Than 014:06
- If Each 0 Equal 114:23
- Modular Example - Function14:34
- Trig - Examples16:44
- A Brief History19:02
- Combines Features From Simula 67 and C19:20
- 1998 - First International Standard Known as C++9820:14
- 2011- New C++1120:24
- C=++ Advantages20:36
- Combines Functional Programming with Object-Oriented Programming21:29
- Compiled Code Works Closely with the Hardware22:18
- Language Elements22:51
- C++ Disadvantages23:09
- Little Run-Time Checking23:39
- Library of Tools are Not as Extensive as Other Languages24:40
- Ready to Get Started!26:35
Getting Started
44m 24s
- Intro0:00
- Overview0:15
- Acquiring a C++ Compiler - Windows1:06
- Setting up MinGW2:44
- Acquiring a C++ Compiler - Others3:24
- Redhat (Fedora) Linux3:40
- Ubuntu (Debian) Linux4:19
- Apple Macintosh4:43
- Acquiring a C++ Compiler - IDE5:05
- Eclipse CDT5:12
- Eclipse Older Version6:30
- Acquiring an Editor6:46
- Acquiring an Editor8:36
- Linux8:40
- Macintosh8:51
- 'Hello World' Everybody's First Program9:35
- Source Code13:31
- Basic Program Structure17:50
- Example20:14
- Basic Program Structure21:02
- Main Function Definition21:29
- Compiling, Linking, Running22:14
- Examples: Compile22:47
- Link24:55
- Build Tools26:11
- Build a 'Makefile'26:26
- Integrated Development Environment (IDE)27:25
- Quick Note on Variable Declaration28:03
- Example: Integers28:26
- Example: Floating Point Numbers29:05
- Your Second Program - User Input Basic 'How Old Are You?'30:49
- Workspace / Source Code32:21
- New Workspace33:29
- Quick Note on Character Arrays34:44
- Example35:34
- Your Third Program - User Text Input 'What’s Your Name?'37:13
- Example37:46
- Workspace39:50
- Do Your Homework!42:56
Data Storage
10m 8s
- Intro0:00
- Overview0:12
- Variables, Identifiers, Names1:34
- Identifiers Defined1:51
- Example of Valid Identifiers2:16
- Styles: Multi-Word Identifiers3:59
- Compiler Doesn't Care4:51
- Styles: Identify Identifiers5:38
- Simple Data Types8:08
- Variable Type Declaration10:35
- Commentary11:42
- More Than One Variable Declared13:22
- Compiler Allocates Storage14:23
- Example15:30
- Variable Initialization16:47
- C Style17:39
- Example18:23
- C++ 'Class' Style18:35
- Numeric Literals19:41
- 'Long' Types21:15
- Beware: Do Not Use23:40
- Character Literals24:27
- Examples24:49
- Quoted Special Characters27:43
- String Literals29:55
- String Object32:28
- Homework33:20
Operators & Expression
32m 24s
- Intro0:00
- Overview0:11
- Parameters for Operators0:47
- Left Side vs. Right Side1:56
- Example3:12
- Operators - Assignment3:40
- Assignment - Don't Confuse Readers5:17
- Operators - Arithmetic7:53
- Operators - Compound Assignment9:38
- Operators: Increment / Decrement10:24
- Examples10:50
- Pre- vs. Post-Operator11:20
- Operators - Comparators12:19
- Operators - Logical13:38
- Examples14:35
- Operators - Boolean Truth Tables15:45
- Examples16:55
- Operators - Input and Output18:42
- I/O Stream18:56
- Operators - Type Casting, Sizeof20:42
- 'Sizeof'21:46
- Type-Casting Cautions22:27
- Classic Example22:38
- Type Promotion25:46
- Operator Precedence26:58
- Firth Things: Unary Operators27:27
- Expressions28:32
- Examples28:58
- Cautions30:17
- Classic Example30:31
- Hey! Wrong Kind of Operator!32:13
Branching
26m 15s
- Intro0:00
- Overview0:03
- Code Blocks, Scope1:32
- 'Scope' of the Block of Code2:27
- Scope Example2:56
- 'If' Statement5:20
- 'Else' Clause6:43
- Example7:01
- Nested 'If'7:52
- Example8:08
- More Nested 'If'8:41
- 'If' Without Blocks9:53
- 'Chained If'10:35
- Example10:51
- 'If' Statement's Expression11:36
- 'If' Statement Stylistic Notes12:55
- 'Else' Clause Stylistic Notes14:24
- 'Switch' Multiple Choice15:15
- 'Switch' Like 'Chained-If'16:35
- 'Switch Tricks'17:56
- Leave Break Out18:10
- 'Conditional' Statement Cautions19:54
- 'Conditional' Statement Example21:56
- Another Example23:05
- 'Goto' and 'Label'23:40
- Do's and Don'ts1:54
Looping
28m 19s
- Intro0:00
- Overview0:08
- Important Kind of Branch1:17
- Branch That Loops1:43
- In The Beginning3:51
- 'While' Loop - Pre-Test4:45
- 'Do-While' Loop - Post-Test6:47
- 'Do-While' Run It Again7:31
- 'For' Loop-Counting Loops9:38
- 'For' Loop-Most Typical Use11:46
- Useful Feature12:47
- 'For' Loop - Similar to 'While'13:47
- Example13:57
- Equivalent 'While' Loop14:34
- 'Break' Out of Loop15:50
- 'Break' Examples17:07
- 'Continue' Back to Beginning18:49
- 'For' Loop19:04
- 'Continue' Caution20:05
- Infinite Loop20:58
- Loop Inside of Loops21:44
- Typical Bug22:50
- More Looped Loops24:08
- What a Loop!27:46
Functions, Part 1
14m 44s
- Intro0:00
- Overview0:09
- Value of Functions0:46
- Example Without Function2:04
- Example With Function3:30
- Function Prototype4:11
- Return Type4:33
- Name4:40
- Parameters4:52
- Nomenclature5:27
- Prototype Example5:45
- Function Definition6:28
- Definition Example8:11
- Function Parameter Types8:52
- Definition9:47
- Using the Function11:09
- Functions Return Single Value13:15
- Break Problem into Pieces14:12
Functions, Part 2
16m 56s
- Intro0:00
- Overview0:09
- Parameters by Value0:47
- Example1:26
- Parameters by Reference2:55
- Example3:53
- Parameters by Reference Example4:20
- Default Parameters6:36
- Acceptable Example7:13
- Unacceptable Example7:27
- Default Parameters Example7:42
- Return Value8:41
- Special Type: Void10:31
- Overloading11:07
- Name Accordingly & Carefully11:17
- Example11:59
- Overloading Example12:31
- Modularization13:41
- Smaller Modules14:14
- Isolated Modules15:17
- Cut Into More Pieces16:31
Arrays & Pointers
40m 44s
- Intro0:00
- Overview0:10
- Use a 'Chunk' of Memory3:22
- Declaring an Array6:27
- Example6:55
- Select an Element From Array8:44
- Example10:15
- Multi-Dimensional Array12:15
- Examples13:09
- Compile-Time Initialization14:18
- Example15:04
- Multi-Initialization16:27
- Character String Initialization18:27
- Examples18:43
- Pointers20:11
- Example21:02
- Pointer Declaration22:20
- Reference Operator22:52
- Referencing and Dereferencing24:30
- Example24:49
- Pointer Arithmetic27:10
- Function Call-By Reference31:06
- Function Array/ Pointer Example32:21
- Null Terminated Character Arrays34:22
- Example35:13
- 'New' and 'Delete'36:31
- Example37:44
- Array of Pointers40:19
Structures
24m 37s
- Intro0:00
- Overview0:10
- Keep Data Organized1:25
- Multiple Arrays2:01
- Structure Declaration3:19
- Example4:00
- Structure Declaration4:24
- Example4:53
- Structure Usage6:03
- Example Structure6:09
- Accessing Members8:27
- Structure Member Structures9:38
- Example9:50
- Initializing Member Structures11:35
- Example11:44
- Access Data Within Data12:46
- Arrays of Structures13:11
- Multi-Dimensional? Of Course!15:18
- Pointers to Structures17:55
- Pointed-to Structure Members21:22
- Structure Pointer Operator22:41
- Example22:53
- Structure for Pointers24:16
Input/ Output (IO)
43m 36s
- Intro0:00
- Overview0:15
- Character Input Streams3:02
- 'Getline' Method5:12
- Input Stream Object Methods7:47
- Input Examples11:56
- Character Output Streams15:19
- 'Cout' = Standard Output15:34
- 'Cerr' = Error Output16:32
- Insertion Operator17:45
- Format18:12
- Output Stream Object Methods18:23
- Output Examples21:23
- Formatting: I/O Manipulators23:38
- Set the Width24:04
- More I/O Manipulators28:20
- Scientific28:44
- Set Precision29:15
- Examples31:04
- File Input/Output32:13
- Output Streams33:52
- Insertion Operator35:15
- Example35:22
- Input Streams36:50
- Example37:35
- Error Messages38:18
- 'errno'39:10
- Example39:49
- File Example41:06
- Got Files?42:58
Review Using Real Code
48m 26s
- Intro0:00
- Overview0:19
- Checkbook Program1:15
- Source Code1:26
- 3 Structures1:47
- Checkbook Program6:36
- Create Functions7:15
- Source Code: Constant Set up / Version7:45
- Delimiter9:25
- Load Transactions13:00
- Dump Transactions13:17
- Utility Functions14:56
- Structures16:14
- Bank Structure16:35
- Transactions16:44
- Global Constants17:00
- Source Code: Global Variables18:29
- Function Prototypes18:52
- Source Code19:36
- Find the Bug in the Code22:04
- Function Prototypes24:32
- 'Main', the Director25:16
- Source Code25:44
- Read Initial Data26:13
- Source Code26:45
- Function to Create 'Init' File30:34
- Transactions File Functions35:12
- User Action Functions36:58
- First User Action37:10
- Source Code38:32
- Utility Functions44:34
- 'Stringstream'45:23
- Write Your Own Cheque47:26
C++ Standard Library
27m 53s
- Intro0:00
- Overview0:22
- System Time1:42
- Example2:48
- Back to the Future!3:31
- Human-Readable Time5:27
- Example5:47
- Static Memory6:36
- Local and GMT Time7:45
- localtime8:28
- gmtime8:42
- Example8:50
- Time Structure9:23
- Formatting Time10:16
- Example11:02
- Math Library11:58
- Other Math Functions13:11
- Functions from cstdlib (Standard Library)13:47
- More from cstdlib15:31
- Functions from ctype19:29
- More From ctype21:27
- Example21:55
- Functions from cstrings23:00
- More from cstrings25:16
- Additional Length Limited Versions25:46
- See the Library!27:30
Handling Strings
33m 1s
- Intro0:00
- Overview0:09
- Two Kinds of Strings2:21
- Object String from C++2:25
- Classic Strings from C3:30
- C++ String Object4:24
- Useful Member Functions4:34
- String Object Examples7:35
- Initialization7:39
- Operations7:49
- String Object Member Functions10:27
- Member Function10:40
- Example11:02
- More Member Functions13:34
- Arrays of String Objects16:18
- Examples16:34
- Classic C Character String Array19:00
- Defined As19:24
- Useful Functions for C-String Operations20:20
- Array of Classic C String22:00
- Examples22:10
- Some Reasons Against Classic24:36
- Examples24:59
- Need to Use String Functions25:33
- Cautions with Classic C Strings26:11
- Examples26:23
- Especially with Copying and Concatenation27:16
- But You Can't be Rid of It29:35
- Examples30:00
- Don't Tangle Your Strings!32:30
Object Oriented Programming
22m 45s
- Intro0:00
- Overview0:15
- Concepts1:53
- Object Oriented Programming (OOP)2:42
- Some Terminology3:34
- Object Defined3:39
- Class Defined4:07
- Instance Defined4:20
- Example4:35
- Abstraction5:21
- Emphasis on Essential5:36
- Emphasis on Characteristics6:17
- Encapsulation7:29
- Private Data8:16
- Public Methods8:40
- Protected Data9:14
- Inheritance10:08
- Example10:39
- Polymorphism11:39
- Example: Operator Overloading12:24
- 'Is-a' vs. 'Has-a'14:00
- Multiple Inheritance15:07
- Example15:28
- Diamond Problem17:59
- Constructors / Destructors18:27
- My Kind of Inheritance22:25
Source Files & OO Samples
35m 58s
- Intro0:00
- Overview0:19
- Source File Separation2:31
- Compilation Units4:24
- Example4:34
- Header File (Interface)7:23
- Include Header File9:25
- Example10:12
- Protect from Multi-Include11:42
- Example12:33
- Source File (Implementation)14:05
- Algorithms14:23
- Commands15:49
- Class Syntax17:25
- Private Member18:00
- Protected Member18:23
- Public Member18:52
- Class Prototype for Header21:13
- Class Implementation23:21
- Controlled Access24:49
- Inheritance25:44
- Implementation27:44
- Virtual Method29:04
- Pointer31:37
- Virtual Example32:02
- Base Class32:12
- Derived Classes32:25
- Polymorphic Example33:14
- Finished35:23
Loading...
This is a quick preview of the lesson. For full access, please Log In or Sign up.
For more information, please see full course syllabus of Introduction to C++
For more information, please see full course syllabus of Introduction to C++
Introduction to C++ Functions, Part 2
Lecture Description
In this lesson, our instructor Alvin Sylvain gives an introduction to part two of functions. He starts by discussing the parameters by value and reference, as well as default parameters. He then moves on to return value, overloading and modularization.
Bookmark & Share
Embed
Share this knowledge with your friends!
Copy & Paste this embed code into your website’s HTML
Please ensure that your website editor is in text mode when you paste the code.(In Wordpress, the mode button is on the top right corner.)
×
Since this lesson is not free, only the preview will appear on your website.
- - Allow users to view the embedded video in full-size.
Next Lecture
Previous Lecture
2 answers
Thu Jun 25, 2015 6:55 PM
Post by Alex Moon on June 24, 2015
Hello, I don't understand what is being asked here.
2. Write a function that takes a float parameter, and uses it as the divisor into two, then subtracts two. That is, do the following arithmetic: (2.0 / parameter + 2.0)
Return the result in the parameter, but have a Boolean return value of “false†if the arithmetic can not be performed because the parameter is too small (we want to avoid getting overflow errors or divide-by-zero errors), or “true†otherwise. You will have to test the parameter before performing the operation.
Thus, if the function returns “trueâ€, the parameter has been changed to its new value, and the caller may use it. Otherwise, it remains unchanged, and the return value tells the caller the parameter can not be used.
In "Return the result in the parameter, but have a Boolean return value of “false†if the arithmetic can not be performed ... or “true†otherwise"
is it telling me to create a function that can either return a boolean AND a float result?
Should the program print the value passed by reference in parameter? Thank you
2 answers
Last reply by: Timothy White
Fri Oct 31, 2014 7:42 AM
Post by Timothy White on October 30, 2014
Not necessarily related to this lecture, but is it possible to use C++ in a webpage? This is the first language I have learned that is not geared toward web development, so I wanted to know if there is a way to do so.
1 answer
Thu Jan 10, 2013 2:56 PM
Post by Karpis Sanosyan on January 10, 2013
Hello I have love the lessons and they really help, but i have a quick question. Its not as much as related to the lessons but to educator.com it's self. Could you please possible make an android and iPhone app for this site. Because I find that I don't always have my computer when I'm on the go and want to watch the lectures.
1 answer
Sun Oct 28, 2012 7:44 PM
Post by David McNeill on September 30, 2012
Alvin I hope you could help me move on to the next section in my course.
when doing homework for this section "functions part 2" i got rather confused on question 2. After struggling to understand the problem i came up with a solution but i don't know if it is the correct solution. Please help me move on so i know i understand what i have learned up until this point. here is my solution please let me know if I have understood your question:
//============================================================================
// Name : Function_Return_Boolean.cpp
// Author : David K McNeill
// Version : 1.0
// Copyright : Your copyright notice
// Description : checks a parameter is not to small before calculating and assigning the result to a variable.
//============================================================================
#include <iostream>
using namespace std;
// Function that checks if a parameter is not to small to divide.
// If parameter is not to small this function will perform the following arithmetic:
// 2.0 / parameter -2; it will then pass out the result to a parameter and return the
// value true to the caller.
// if the parameter is to small then it will return false to the caller.
bool divide_Add (float para, float & result, bool & a) {
//result = (2.0 / para -2);
if (para == 0) {
a = false;
}
else {
result = (2.0 / para -2);
a = true;
}
return a;
}
//global variables.
float para = 10; // parameter to be checked if its to small.
bool test = true; // variable that is assigned the function boolean return value.
bool a = false; // variable that determines what value the function returns.
float actual = 0; // variable we want to assign the function arithmetic to if all is Ok.
float result = 0; // variable that we assign the value of function arithmetic.
// main function
int main() {
test = divide_Add (para, result, a); // this is the call to the function
if (test == true) { // if function returns true we will assign the function arithmetic to are variable.
actual = result; // and print out "yippe it worked" as well as the value of 'actual', 'result' and 'para'.
cout << "Yippe it worked."<<endl;
cout << actual <<endl;
cout << result <<endl;
cout << para <<endl;
}
else {
cout << "number to small to use." <<endl; // if function returns false the this warning will be printed
} // and no value assigned to are variable.
return 0;
}
0 answers
Post by Alex Abraham on August 19, 2012
In the first example, the code is more broken than you think :p
The first time, it takes 7, adds 7, and gets 14.
The second time, it takes 14, adds 14, and gets 28.
The third time, it takes 28, adds 28, and gets 56.
One way to fix this error is to say something like: number = second; before the for statement and change the command in the for statement to number += second;
Also at the end it should say return number;